The full series of articles consists of
- Content (this article)
- URLs - how to optimise your URLs for search
- Indexing - mechanisms that help to ensure the correct parts of your site are found by search engines
Quality Content
If you want your site to rank well over the long term, the importance of high quality original content cannot be overstated. If your content provides value to your target audience, they will want to link to it and share it via social media and other avenues. The more good quality links you get, the better you will be ranked. There is no real shortcut to this, but there are some technical ways in which you can present your content so that it is more search engine friendly. However, I cannot stress enough how important it is to keep your user foremost in mind when creating content for your site.
Title and Metatags
Every page should have a title. This is set in the <title>
element within the <head>
section of the page. The title is displayed in search engines result pages (SERPs). The title should be unique, descriptive and accurate. The title should contain keywords that reflect the page content, and it should be easy to read. There are no hard and fast rules in terms of length, but you will often see recommendations to keep the character count below 60. This is mainly to accommodate what is displayed in SERPs, but any additional characters are processed as part of the ranking system.
Setting the title - Web Forms and MVC
If you are developing with Web Forms, there are a number of ways to set the page title. You could hard code it in the Master page's HTML, but doing that will result in every page in your site having the same title. If you want a unique and descriptive title per page, you will set the title at the page level. If the title is static, you can set it in the aspx file in the @ Page
directive:
<%@ Page Title="About my site" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true" CodeBehind="About.aspx.cs" Inherits="WebFormsSEO.About" %>
If you want to set a dynamic title, for example you might want to use the title of your blog article or recipe depending on your site content, you can do this from code-behind by setting the Page.Title
(or this.Title
or just Title
) value. The approach you take to setting the value depends on the source of the data being used to construct your title. Most often, you will use a control's DataBound
or RowDataBound
event handler to access the control's data source and extract whatever values you want. Whichever way you choose, you should be aware of the order of precedence that governs what value eventually appears.
Whatever you hard code into the HTML of a Master Page will always appear. The standard Web Forms site template makes use of this feature by hard coding " - My ASP.NET Application" as part of the <title>
value, but it also allows the first part of the <title>
to be set dynamically:
<title><%: Page.Title %> - My ASP.NET Application</title>
When you add a new aspx file to your application, the default value for Title
in the @ Page
directive is an empty string:
<%@ Page Title="" Language="C#" MasterPageFile="~/Site.Master"
If you leave it like that, no matter where else you try to set the Page.Title
value, it will stay as an empty string. So unless you want to hard code a value in the @ Page
directive, you should delete the Title
entry from it. Assuming you don't leave this value as an empty string, anything you set in the @ Page
directive will be overwritten by whatever you set in the page's code-behind. And this in turn will be overwritten by anything that you set from the Master Page's code-behind.
The MVC template also includes some default mark up to cater for the value of the <title>
element in the layout page:
<title>@ViewBag.Title - My ASP.NET Application</title>
You can manage the value of ViewBag.Title
by setting it in the controller. You can also set it in the content page:
@{ ViewBag.Title = "Home Page"; }
This will override any value set in the controller. Note, since ViewBag
is a dynamic object, instead of using Title
as the property name, you can just as easily use PageTitle
, or indeed anything you like.
Meta Descriptions
Meta descriptions are your opportunity to advertise your page on SERPs. They are set using the HTML <meta>
tag:
<meta name="description" content="This is where the description goes" />
This should be used to provide a description of the page content. It should include key words relevant to the content and be written in natural language i.e. not a list of keywords. The meta description may be used by most search engines in the SERPs to let searchers know what each result is about.
The image above illustrates how Google uses the meta description (highlighted in yellow) in a search result for "sending email aspnet mvc". Google won't always use your meta description, and certainly won't use all of it if the total length exceeds about 160 characters. The text that Google provides will be taken from the page content itself, if it feels that is more appropriate than the content of the meta description. In this particular case, my description exceeds the 160 limit by quite a way, and the amount of characters that Google chose to use was further limited by their decision to include the date of the article at the beginning of the description.
Prior to the introduction of ASP.NET 4, you would use a ContentPlaceholder and the HtmlMeta class to set a meta description in a Web Forms site. Since ASP.NET 4, the Page
class has included a MetaDescription
property which enables Web Forms developers to set this value easily form code-behind:
public partial class About : Page { protected void Page_Load(object sender, EventArgs e) { MetaDescription = "A page that describes what we are about"; } }
MVC developers can use a similar approach to setting the title, by using a placeholder in the layout page and ViewBag
to pass a description dynamically:
<meta name="description" content="@ViewBag.Description">
In my case, I have a field in the database for the introductory paragraph of each article, and another for the meta description. If I haven't populated the description field, the first paragraph is used as the content for the meta description:
ViewBag.Description = article.Description != null ? article.Description : article.Introduction;
Meta Keywords
It's generally recognised these days that meta keywords serve no useful purpose at all. Search engines by and large completely ignore them. Nevertheless, some developers still like to include them, and ASP.NET 4 introduced a MetaKeywords
property for the Page
class along with the MetaDescription
property:
protected void Page_Load(object sender, EventArgs e) { MetaKeywords = "spam, spam, spam"; }
Structured Data / Rich Snippets
Google will sometimes attempt to extract some meta data from a search result to enhance entries within the SERPs listings. The previous image shows the article date being included as part of the description. Other search results can include much more structured data in the form of what Google refers to as "Rich Snippets" as the next image showing a search result for "christmas cake recipe" illustrates (the highlighting is mine):
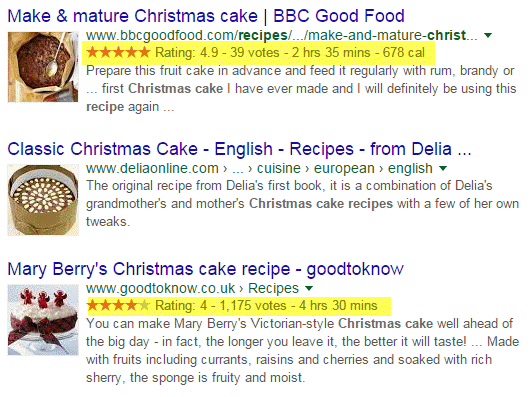
Along with the description, the result also shows an image, rating, total time required to prepare and cook the cake, and in the first result's case, the calorie value of the recipe . I'm sure the Google engineers are clever enough to be able to parse this data from web pages using Natural Language Processing (or whatever alpha-geek fields they work in), but their job is made much easier by the use of structured data by the web page authors. Having your listing enhanced like this could lead to higher click through rates.
The structured data vocabulary that Google and other search engines recognise is described at schema.org. A wide variety of entities are catered for including such things as technical articles, music events, medical procedures, child care businesses and so on, The vocabulary is applied to web pages in one of two ways: either as special attributes to HTML elements; or as JSON-LD. If you choose to use attributes, they can be applied using one of two alternative encodings, Microdata or RDFa. I use Microdata in my site, so that's the format I shall explore before taking a look at JSON-LD.
Microdata
Typically, an identifiable section of a page will be devoted to the item you want to mark up. It might be the whole page, or just some part of it. You use the itemscope
attribute to define the area that encloses the information relating to the item, and itemtype
to specify the actual type of item you are describing. The value of the itemtype
attribute is a URL pointing to the schema for the specific item. A technical article might be enclosed in a div
or an HTML5 article
tag.
<article itemscope itemtype="http://schema.org/TechArticle">
Thereafter, you use attributes to describe the item-specific properties according to the itemtype
under focus. In the case of a technical article, these item might include articleBody
, author
, genre
and so on. Google specifies required properties for certain items for the markup to be valid. In the case of an article, Google requires a headline
, datepublished
and image
. Individual properties are marked up using the itemprop
attribute:
<h1 itemprop="headline"> @Model.Article.Headline </h1>
You can provide additional information that isn't visible to users via meta tags. For example, you can provide a facility for users to rate content, and the rating itself is represented by an AggregateRating item. You can tell search engines and other parsers what the highest and lowest possible values for your rating system are as follows:
<meta itemprop="bestRating" content="5" /> <meta itemprop="worstRating" content="1" />
JSON-LD
JSON-LD (Javascript Object Notation for Linked Data) is declared either in script tags or a separate .js file. Unlike Mocrodata attributes, it allows the developer to provide the meta data about their content in one place , rather than intermingled with the content itself. Here's how a typical article with rating might appear in a Razor view:
<script type="application/ld+json"> "@@context": "http://schema.org", "@@type": "TechArticle", "headline": "@Model.Article.Headline", "image": [ "image1.jpg" ], "datePublished": "@Model.Article.DateCreated.ToString("T"), "description": "@Model.Article.Description", "articleBody": "@Model.Article.Maintext", "aggregateRating": { "@@type": "AggregateRating", "ratingValue": "@Model.ArticleRating.AverageRating", "reviewCount": "@Model.ArticleRating.TotalRaters" } </script>
The context and type properties are prefixed with single literal @
signs, but in the Razor view, this has to be escaped which is why they are doubled. You only need one @
sign in a Web Forms aspx file:
<script type="application/ld+json"> "@context": "http://schema.org", "@type": "TechArticle", "headline": "<%: Model.Article.Headline %>" ...
Summary
This article looked at some ways in which you can present the content of your ASP.NET site to improve its visibility and meaning to search engines, and potentially increase your click through rates. It covered the importance of the standard HTML title and meta description tags and how to use them. The article then explored how to get your search listing enhanced via the use of structured data and showed two ways in which structured data can be used.
The next article in the series will review the importance that search engines place on URLs and how to optimize them for best SEO results.